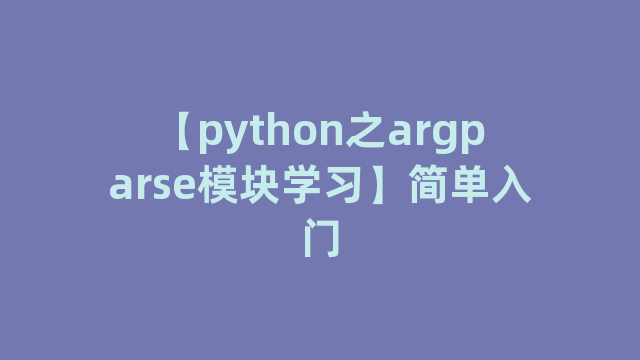
目录
- 0 前言
- 1 入门程序
- 2 参数
-
- 2.1 位置参数
- 2.2 可选参数
- 2.3 矛盾选项
- 3 总结
- 4 参考文献
0 前言
1 入门程序
import argparse parser = argparse.ArgumentParser() parser.parse_args()
$ python3 prog.py
$ python3 prog.py –help usage: prog.py [-h] options: -h, –help show this help message and exit
$ python3 prog.py –verbose usage: prog.py [-h] prog.py: error: unrecognized arguments: –verbose
$ python3 prog.py foo usage: prog.py [-h] prog.py: error: unrecognized arguments: foo
2 参数
2.1 位置参数
import argparse parser = argparse.ArgumentParser() parser.add_argument("square", help="display a square of a given number", type=int) args = parser.parse_args() print(args.square**2)
$ python test.py usage: test.py [-h] square test.py: error: the following arguments are required: square
$ python test.py 4 16
$ python test.py four usage: test.py [-h] square test.py: error: argument square: invalid int value: 'four'
$ python test.py square 4 usage: test.py [-h] square test.py: error: argument square: invalid int value: 'square'
$ python test.py -h usage: test.py [-h] square positional arguments: square display a square of a given number optional arguments: -h, –help show this help message and exit
2.2 可选参数
import argparse parser = argparse.ArgumentParser() parser.add_argument("square", help="display a square of a given number", type=int) parser.add_argument("–verbosity", help="increase output verbosity") args = parser.parse_args() if args.verbosity: print("the square of " + str(args.square) + " is " + str(args.square**2)) else: print(args.square**2)
$ python test.py 4 –verbosity 1 # 效果等同 python test.py –verbosity 1 4 the square of 4 is 16
$ python test.py 4 16
$ python test.py 4 –verbosity usage: test.py [-h] [–verbosity VERBOSITY] square test.py: error: argument –verbosity: expected one argument
$ python test.py -h usage: test.py [-h] [–verbosity VERBOSITY] square positional arguments: square display a square of a given number optional arguments: -h, –help show this help message and exit –verbosity VERBOSITY increase output verbosity
import argparse parser = argparse.ArgumentParser() parser.add_argument("square", help="display a square of a given number", type=int) parser.add_argument("-v", "–verbose", help="increase output verbosity",action="store_true") args = parser.parse_args() if args.verbose: print("the square of " + str(args.square) + " is " + str(args.square**2)) else: print(args.square**2)
$ python test.py -v 4 the square of 4 is 16
$ python test.py 4 -v the square of 4 is 16
2.3 矛盾选项
import argparse
parser = argparse.ArgumentParser() group = parser.add_mutually_exclusive_group() group.add_argument("-v", "–verbose", action="store_true") group.add_argument("-q", "–quiet", action="store_true") parser.add_argument("x", type=int, help="the base") parser.add_argument("y", type=int, help="the exponent") args = parser.parse_args() answer = args.x**args.y
if args.quiet: print(answer) elif args.verbose: print(f"{args.x} to the power {args.y} equals {answer}") else: print(f"{args.x}^{args.y} == {answer}")
$ python test.py 4 2 4^2 == 16
$ python test.py -q 4 2 16
$ python test.py -v 4 2 4 to the power 2 equals 16
$ python test.py -v -q 4 2 usage: test.py [-h] [-v | -q] x y test.py: error: argument -q/–quiet: not allowed with argument -v/–verbose
$ python test.py -vq 4 2 usage: test.py [-h] [-v | -q] x y test.py: error: argument -q/–quiet: not allowed with argument -v/–verbose
3 总结
4 参考文献
神龙|纯净稳定代理IP免费测试>>>>>>>>天启|企业级代理IP免费测试>>>>>>>>IPIPGO|全球住宅代理IP免费测试